Convert CSV file to JSON in Logic app.
This is the full step-by-step process, and I have provided its output below.
I used the below CSV file, separated by pipes ('|'), to test this.
Please follow the below steps.
Step 1: I fetched the file from Blob by searching for the blob and selecting the 'Get blob content' action.
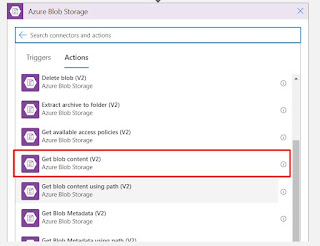
Step 2: For this action, I provided the storage account name and file path.
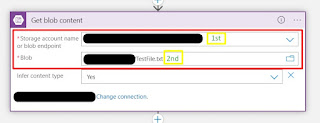
Step 3: To process the output content from the blob, I used the 'Compose' action and applied the 'Split' function.
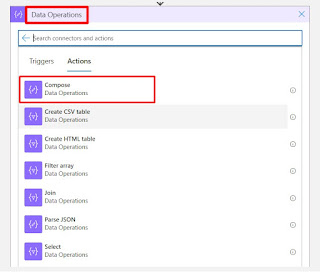
Expression:
split(body('Get_blob_content_'),decodeUriComponent('%0D%0A'))
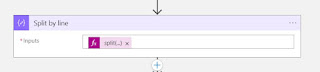
Step 4: Additionally, I used another 'Compose' action to remove the last line, as it appeared empty. You can choose to include or omit this step based on your file structure.
Expression:
take(outputs('Split_by_line'),add(length(outputs('Split_by_line')),-1))

Step 5: Another 'Compose' action was selected to split the text data based on the separator '|'.
Expression:(separator '|')
split(first(outputs('Split_by_line')), '|')
Step 6: Under data operations, I chose 'Select.'
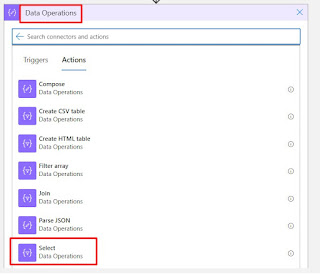
- For the 'From' node, I used the specified expression
skip(outputs('Remove_last_line'), 1)
- For the 'Map' node, I used the provided expression. (separator '|')
Left Right
outputs('Fields')[0] --- split(item(), '|')?[0]
outputs('Fields')[1] --- split(item(), '|')?[1]
outputs('Fields')[2] --- split(item(), '|')?[2]
outputs('Fields')[3] --- split(item(), '|')?[3]
Step 7: Finally, I selected the 'Parse JSON' action and provided the content from the outputs of the 'Select' step.
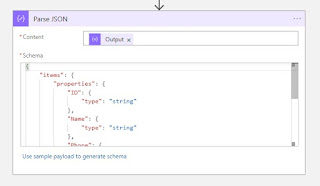
Outputs :
Step 2 output:
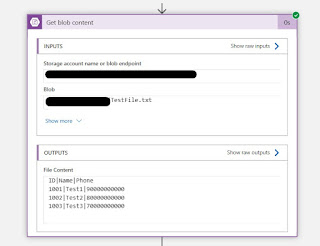
Step 3 Output :
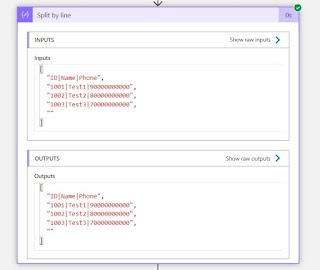
Step 4 Output :
Step 5 Output :
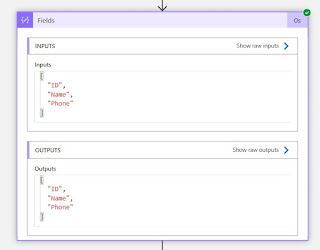
Step 6 Output :
Step 7 Output :
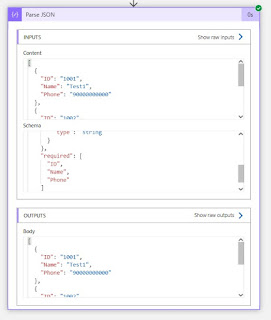
Reference: Link 1, Link 2
Keep Daxing!!